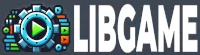 |
LibGame
v0.4.0
The LG Game Engine - Copyright (C) 2024-2025 ETMSoftware
|
18 #ifndef _ISOC11_SOURCE
19 #define _ISOC11_SOURCE
22 #define LIBGAME_NAME "LibGame"
23 #define LIBGAME_V_NUM "0.4.0~beta-20250619"
24 #define LIBGAME_COPYRIGHT_STR "Copyright (C) Emmanuel Thomas-Maurin 2012-2025 - All rights reserved"
26 #define LIBGAME_WEBSITE "https://www.etmsoftware.com"
27 #define LIBGAME_DOWNLOAD_WEBSITE LIBGAME_WEBSITE "/download.php"
28 #define LIBGAME_SUPPORT_WEBSITE LIBGAME_WEBSITE "/help.php"
31 #if !defined(ANDROID_V) && !defined(WIN32_V)
57 #include <android/log.h>
62 #include <SDL_image.h>
63 #include <SDL_mixer.h>
65 #include <SDL_syswm.h>
67 #include <SDL_mouse.h>
71 #include <SDL2/SDL_image.h>
72 #include <SDL2/SDL_mixer.h>
73 #include <SDL2/SDL_ttf.h>
74 #include <SDL_mouse.h>
75 #include <SDL_syswm.h>
78 #include <GLES2/gl2.h>
79 #include <GLES2/gl2ext.h>
80 #include <GLES3/gl3.h>
81 #include <GLES3/gl3ext.h>
86 #define LIBETM_VERBOSE_OUTPUT
90 #ifdef LIBETM_DEBUG_OUTPUT
91 #ifndef LIBETM_VERBOSE_OUTPUT
92 #define LIBETM_VERBOSE_OUTPUT
97 #include "../extra_libs/libetm-0.5.1/libetm.h"
100 #include <libetm/libetm.h>
102 #include "../extra_libs/libetm-0.5.1/libetm.h"
108 #define INFO_ERR INFO_ERR2
113 #define VERBOSE_OUTPUT
117 #ifndef VERBOSE_OUTPUT
118 #define VERBOSE_OUTPUT
122 #define TMPSTR_SIZE ((4 * 1024) - 1)
123 #define FILE_NAME_MAXLEN ((2 * 1024) - 1)
125 #ifdef LIBGAME_COMPILE_TIME
126 #include "../extra_libs/math_3d.h"
127 #include "../extra_libs/stb_image.h"
128 #include "../extra_libs/ufbx.h"
130 #include <libgame/extra_libs/math_3d.h>
131 #include <libgame/extra_libs/stb_image.h>
132 #include <libgame/extra_libs/ufbx.h>
138 #include "lg_shader_progs.h"
139 #include "lg_vertex.h"
140 #include "lg_gr_func.h"
141 #include "lg_textures.h"
142 #include "lg_dds_loader.h"
143 #include "lg_astc_loader.h"
144 #include "lg_string.h"
145 #include "lg_sprites.h"
146 #include "lg_background.h"
148 #include "lg_keyboard.h"
149 #include "lg_mouse.h"
150 #include "lg_touchscreen.h"
151 #include "lg_audio.h"
152 #include "lg_dirs_stuff.h"
153 #include "lg_quaternions.h"
156 #include "lg_3d_primitives.h"
157 #include "lg_opengl_2d.h"
159 #include "lg_camera.h"
160 #include "lg_obj_parser.h"
161 #include "lg_light.h"
162 #include "lg_scene_graph.h"
163 #include "lg_scene.h"
164 #include "lg_collision_detect.h"
165 #include "lg_admob.h"
166 #include "lg_vao_gl_ext.h"
167 #include "lg_cubemap.h"
168 #include "lg_error.h"
169 #include "lg_file_ops.h"
170 #include "lg_android_assets.h"
171 #include "lg_render.h"
172 #include "lg_terrain.h"
173 #include "lg_perlin_noise.h"
174 #include "lg_landscape.h"
175 #include "lg_fbx_parser.h"
176 #include "lg_cam_controls.h"
177 #include "lg_part_sys.h"
180 #include "lg_env_instance.h"
181 #include "lg_math_extra.h"
184 LG_OK = LIBETM_LASTERRORCODE + 1,
187 LG_EXTRA_LIB_INIT_ERROR,
190 LG_CREATE_FILE_ERROR,
193 LG_READ_FROM_FILE_ERROR,
194 LG_WRITE_TO_FILE_ERROR,
195 LG_FILE_ACCESS_ERROR,
196 LG_READ_FROM_FILE_EOF,
210 LG_CONTINUE = LG_LASTERRORCODE + 1,
225 #define LG_WIN_WIDTH_MIN 800
226 #define LG_WIN_HEIGHT_MIN 400
228 #define LG_WIN_D_HEIGHT_HACK 2
231 #define LG_REQUESTED_RED_SIZE 5
232 #define LG_REQUESTED_GREEN_SIZE 6
233 #define LG_REQUESTED_BLUE_SIZE 5
234 #define LG_REQUESTED_ALPHA_SIZE 0
235 #define LG_REQUESTED_DEPTH_SIZE 16
236 #define LG_REQUESTED_STENCIL_SIZE 8
238 int lg_init(
int,
int,
const char *,
const char *,
const char *,
const char *,
const char *,
const char *,
const char *);
void lg_engine_fonts_info()
Definition: libgame.c:914
void lg_reset_viewport()
Definition: libgame.c:803
void lg_show_sys_info()
Definition: libgame.c:1039
void lg_enable_mouse()
Definition: libgame.c:812
void lg_swap_fb()
Definition: libgame.c:1007
Rec2Di lg_get_default_viewport()
Definition: libgame.c:785
void lg_set_new_viewport(Rec2Di viewport)
Definition: libgame.c:795
zboolean lg_load_engine_fonts()
Definition: libgame.c:863
void lg_show_extra_sys_info(SDL_Window *w)
Definition: libgame.c:1136
char * lg_get_sdl_win_flags(SDL_Window *w)
Definition: libgame.c:1217
int lg_init(int win_w, int win_h, const char *win_title, const char *app_name, const char *app_cmd, const char *org_name_android, const char *app_name_android, const char *assets_folder, const char *app_wr_folder)
Definition: libgame.c:47
zboolean sdl2_is_installed()
Definition: libgame.c:1197
void lg_quit(int exit_code)
Definition: libgame.c:924
void lg_list_opengl_extensions()
Definition: libgame.c:828
void lg_disable_mouse()
Definition: libgame.c:820
void lg_show_lib_info()
Definition: libgame.c:1015
Definition: lg_gr_func.h:49